Tiếp theo bài viết hôm nay mình sẽ hướng dẫn các bạn cách viết trang php để trả về một array json và dùng volley để lấy dữ liệu, kết hợp thư viện glide để load ảnh một cách đơn giản nhất.
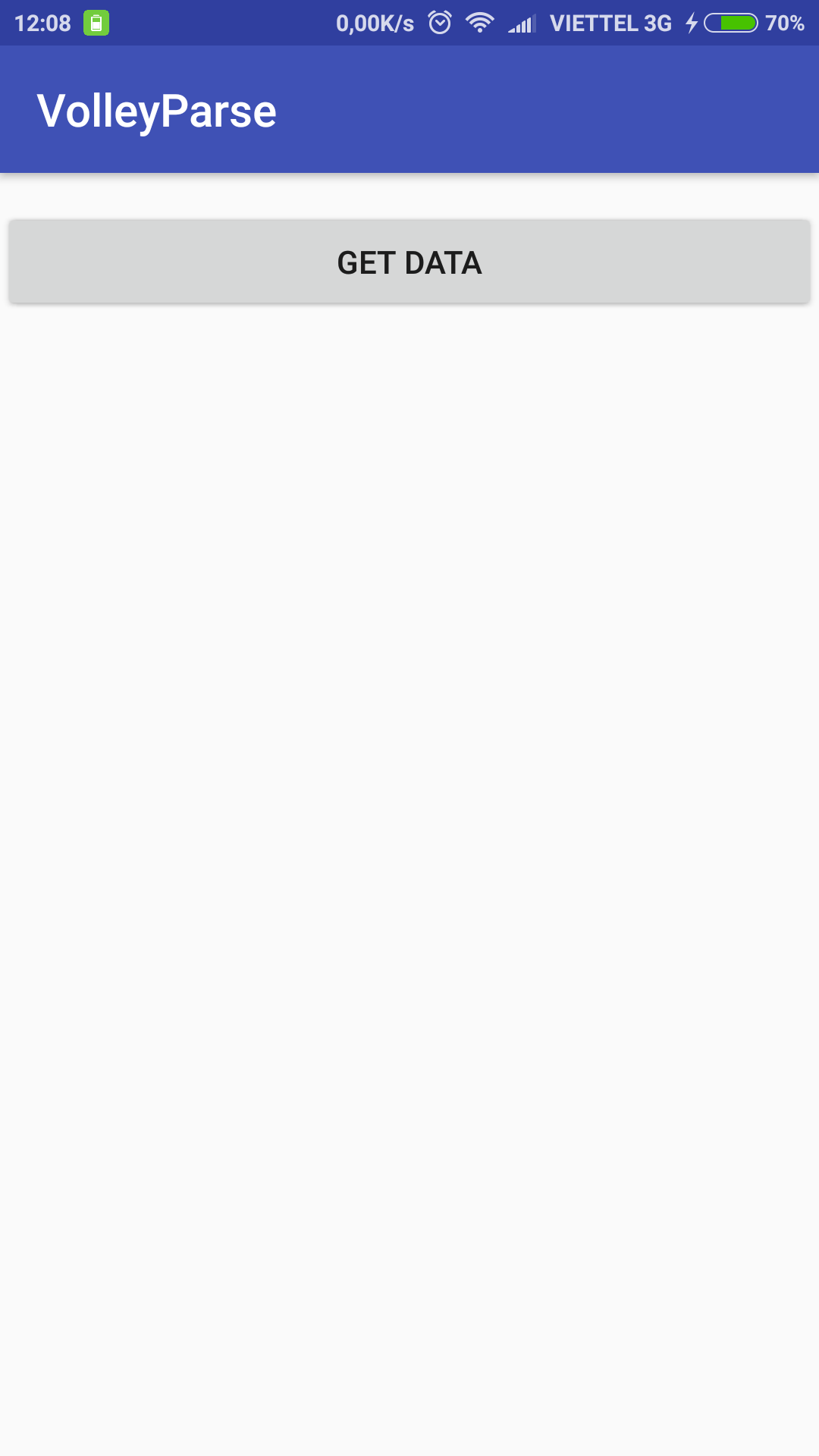
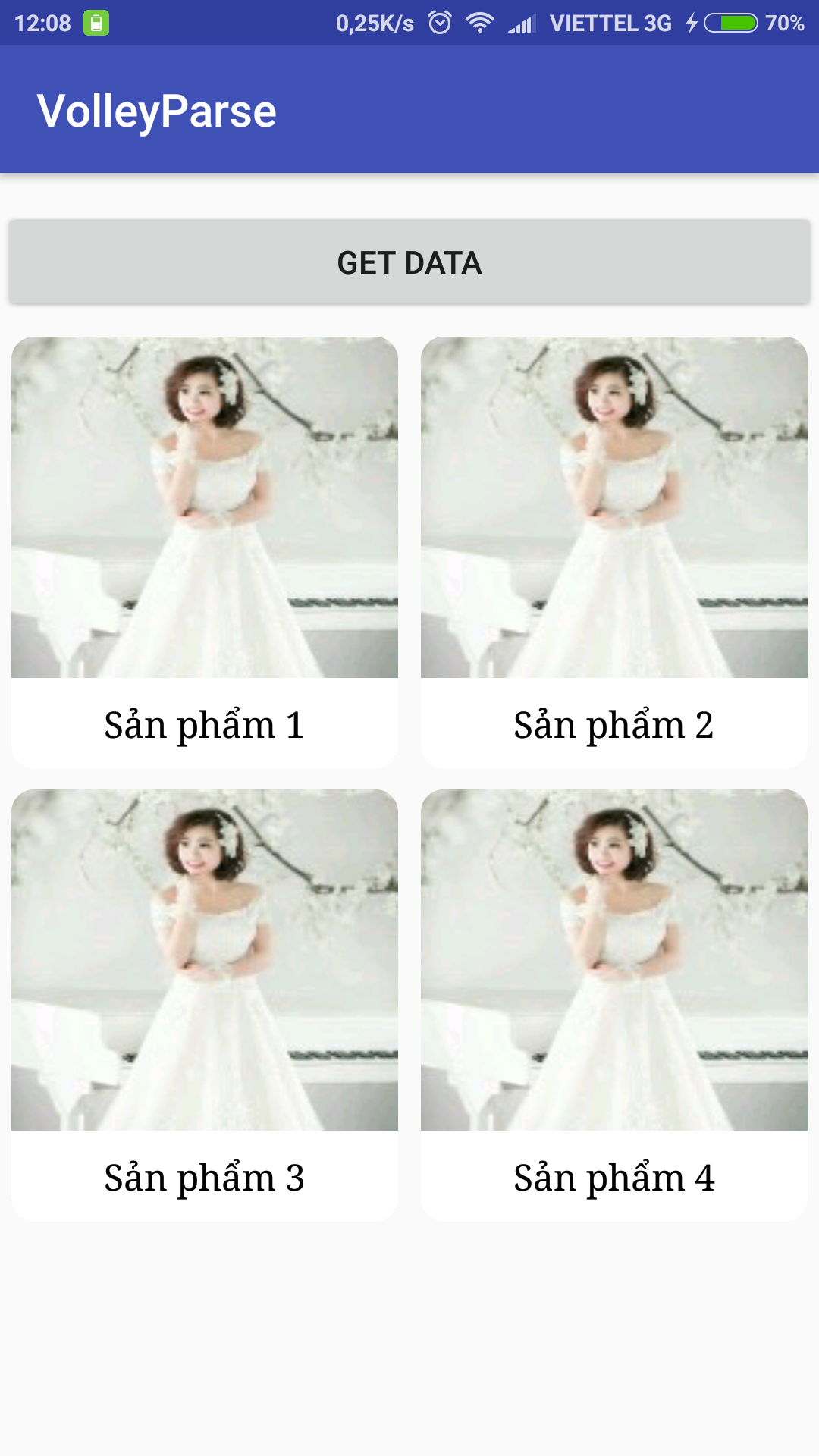
- Các bước thực hiện
– Tạo table, insert dữ liệu trên database
– Viết trang php để lấy dữ liệu
– Sử dụng volley gửi request nhận data
– Parse data lên recyclerView - Các thư viện sử dụng
compile 'com.android.volley:volley:1.0.0' compile 'com.github.bumptech.glide:glide:3.7.0' compile 'com.android.support:recyclerview-v7:24.2.1' compile 'com.android.support:cardview-v7:24.2.1'
Các bước thực hiện
– Tạo bảng và data:
CREATE TABLE `product`( `id_product` INT PRIMARY KEY AUTO_INCREMENT, `product_name` VARCHAR(50) NOT NULL, `decription` VARCHAR(100) NOT NULL, `price` VARCHAR(100) NOT NULL, `thumnail` VARCHAR(200) NOT NULL UNIQUE (id_product) ); INSERT INTO `product`(`product_name`, `decription`, `price`, `thumnail`) VALUES ("Sản phẩm 1","Đây là mô tả của sản phẩm 1","100.000","http://juliettewedding.vn/cdn/store/1339/album/4503/01%20copy3.jpg"); INSERT INTO `product`(`product_name`, `decription`, `price`, `thumnail`) VALUES ("Sản phẩm 2","Đây là mô tả của sản phẩm 2","100.000","http://juliettewedding.vn/cdn/store/1339/album/4503/01%20copy3.jpg"); INSERT INTO `product`(`product_name`, `decription`, `price`, `thumnail`) VALUES ("Sản phẩm 3","Đây là mô tả của sản phẩm 3","100.000","http://juliettewedding.vn/cdn/store/1339/album/4503/01%20copy3.jpg"); INSERT INTO `product`(`product_name`, `decription`, `price`, `thumnail`) VALUES ("Sản phẩm 4","Đây là mô tả của sản phẩm 4","100.000","http://juliettewedding.vn/cdn/store/1339/album/4503/01%20copy3.jpg")
– Viết trang php để lấy dữ liệu:
<?php require_once('dbConnect.php'); mysqli_set_charset($con,'utf8'); $query = "SELECT * FROM `product`"; $result = mysqli_query($con,$query); $output = array(); while($row = mysqli_fetch_array($result)) { $id_product = $row["id_product"]; $product_name = $row["product_name"]; $decription = $row["decription"]; $price = $row["price"]; $thumnail = $row["thumnail"]; array_push($output, new Product($id_product,$product_name,$decription,$price,$thumnail)); } echo json_encode($output); //return json array // Create object class Product{ var $id_product; var $product_name; var $decription; var $price; var $thumnail; function Product($id_product,$product_name,$decription,$price,$thumnail){ $this->id_product = $id_product; $this->product_name = $product_name; $this->decription = $decription; $this->price = $price; $this->thumnail = $thumnail; } } ?>
Upload trang php lên host
Check dữ liệu trả về có thể sử dụng extention postman, hoặc dùng codebeauty
– Tiến hành get dữ liệu về app:
+ activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="15dp" android:id="@+id/button" android:text="@string/btnGet" /> <android.support.v7.widget.RecyclerView android:id="@+id/recycler_view" android:layout_width="match_parent" android:layout_height="match_parent" /> </LinearLayout>
+ grid_item.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:card_view="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="2" android:orientation="vertical"> <android.support.v7.widget.CardView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/cardView" android:layout_weight="0.8" android:layout_marginBottom="0dp" android:layout_marginLeft="5dp" android:layout_marginRight="5dp" android:layout_marginTop="9dp" card_view:cardElevation="0.01dp" card_view:cardCornerRadius="10dp"> <LinearLayout android:id="@+id/top_layout" android:layout_width="match_parent" android:orientation="vertical" android:layout_height="wrap_content"> <ImageView android:id="@+id/img_Thumnail" android:layout_width="match_parent" android:layout_height="99dp" android:scaleType="fitXY" /> <TextView android:id="@+id/tv_Name" android:layout_width="fill_parent" android:layout_height="40dp" android:layout_gravity="bottom" android:textColor="@android:color/black" android:textSize="16dp" android:typeface="serif" android:gravity="center_vertical|center" /> </LinearLayout> </android.support.v7.widget.CardView> </LinearLayout>
+ Tạo một model:
public class Product implements Serializable { private int idProduct; private String productName; private String decription; private String price; private String thumnail; public Product() { } public Product(int idProduct, String productName, String decription, String price, String thumnail) { this.idProduct = idProduct; this.productName = productName; this.decription = decription; this.price = price; this.thumnail = thumnail; } public String getThumnail() { return thumnail; } public void setThumnail(String thumnail) { this.thumnail = thumnail; } public int getIdProduct() { return idProduct; } public void setIdProduct(int idProduct) { this.idProduct = idProduct; } public String getProductName() { return productName; } public void setProductName(String productName) { this.productName = productName; } public String getDecription() { return decription; } public void setDecription(String decription) { this.decription = decription; } public String getPrice() { return price; } public void setPrice(String price) { this.price = price; } }
+ Tạo một Adapter các bạn nào chưa biết sử dụng có thể tham khảo tại đây.
public class ProductAdapter extends RecyclerView.Adapter<ProductAdapter.ShowHolder> { private List<Product> productList; private Context context; class ShowHolder extends RecyclerView.ViewHolder { CardView cardView; TextView tvProductName; ImageView img_Thumnail; ShowHolder(View itemView) { super(itemView); cardView = (CardView) itemView.findViewById(R.id.cardView); tvProductName = (TextView) itemView.findViewById(R.id.tv_Name); img_Thumnail = (ImageView) itemView.findViewById(R.id.img_Thumnail); } } public ProductAdapter(List<Product> productList, Context context) { this.productList = productList; this.context = context; } @Override public ShowHolder onCreateViewHolder(ViewGroup viewGroup, int i) { View view = LayoutInflater.from(viewGroup.getContext()) .inflate(R.layout.gird_item, viewGroup, false); ShowHolder showHolder= new ShowHolder(view); return showHolder; } @Override public void onBindViewHolder(ShowHolder showHolder, int i) { Product product = productList.get(i); showHolder.tvProductName.setText(product.getProductName()); Glide.with(context).load(product.getThumnail()) .override(150,150).centerCrop().into(showHolder.img_Thumnail); } @Override public int getItemCount() { return productList.size(); } }
+ Xử lý lấy dữ liệu tại MainActivity
Dữ liệu nhận về là một mảng array, mình tiến hành chuyển các phần tử thành Object sau đó set giá trị vào List.
JsonArrayRequest request = new JsonArrayRequest(Request.Method.GET, URL_GET_PRODUCT, null, new Response.Listener<JSONArray>() { @Override public void onResponse(JSONArray response) { try { // Convert json array to jsonobject for (int i = 0; i < response.length(); i++) { JSONObject item = response.getJSONObject(i); Product product = new Product(); product.setIdProduct(item.getInt("id_product")); product.setProductName(item.getString("product_name")); product.setPrice(item.getString("decription")); product.setDecription(item.getString("price")); product.setThumnail(item.getString("thumnail")); productList.add(product); } productAdapter.notifyDataSetChanged(); } catch (Exception ex) { Log.e(TAG, ex.toString()); } loading.dismiss(); } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { loading.dismiss(); VolleyLog.e(TAG, error.toString()); } }); RequestQueue requestQueue = Volley.newRequestQueue(MainActivity.this); requestQueue.add(request); }
+ Xử lý sự kiện click đổ dữ liệu vào adapter
// Get Product List getAllProduct(); productAdapter = new ProductAdapter(productList, MainActivity.this); recyclerview.setAdapter(productAdapter);
Toàn bộ code phần xử lý:
public class MainActivity extends AppCompatActivity { private RecyclerView recyclerview; private RecyclerView.LayoutManager mLayoutManager; private ArrayList<Product> productList; private ProductAdapter productAdapter; private Button btnGet; String TAG = MainActivity.class.getSimpleName(); String URL_GET_PRODUCT = "http://dev.androidcoban.com/blog/getallproducts.php?"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); addControl(); addEvent(); } private void addEvent() { btnGet.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // Get Product List getAllProduct(); productAdapter = new ProductAdapter(productList, MainActivity.this); recyclerview.setAdapter(productAdapter); } }); } private void addControl() { recyclerview = (RecyclerView) findViewById(R.id.recycler_view); btnGet = (Button) findViewById(R.id.btnGetData); recyclerview.setHasFixedSize(true); // Create 2 col mLayoutManager = new GridLayoutManager(MainActivity.this, 2); recyclerview.setLayoutManager(mLayoutManager); productList = new ArrayList<>(); } /** * Get all project * result json array */ private void getAllProduct() { final ProgressDialog loading = new ProgressDialog(MainActivity.this); loading.setMessage("Loading...."); loading.show(); JsonArrayRequest request = new JsonArrayRequest(Request.Method.GET, URL_GET_PRODUCT, null, new Response.Listener<JSONArray>() { @Override public void onResponse(JSONArray response) { try { // Convert json array to jsonobject for (int i = 0; i < response.length(); i++) { JSONObject item = response.getJSONObject(i); Product product = new Product(); product.setIdProduct(item.getInt("id_product")); product.setProductName(item.getString("product_name")); product.setPrice(item.getString("decription")); product.setDecription(item.getString("price")); product.setThumnail(item.getString("thumnail")); productList.add(product); } productAdapter.notifyDataSetChanged(); } catch (Exception ex) { Log.e(TAG, ex.toString()); } loading.dismiss(); } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { loading.dismiss(); VolleyLog.e(TAG, error.toString()); } }); RequestQueue requestQueue = Volley.newRequestQueue(MainActivity.this); requestQueue.add(request); } }
Cuối cùng thêm quyền internet vào manifest và chạy project!
Ở project này mình có sử dụng thư viện Glide ở các bài viết sau mình sẽ hướng dẫn các bạn cách khai thác thư viện này, chúc các bạn thành công!